728x90
반응형
1. Attribute Bindings
- HTML 속성 값을 Vue의 상태 속성 값과 동기화되도록 한다.
- v-bind는 생략하고 : 만 쓸 수 있다.
<body>
<div id="app">
<img v-bind:src="imageSrc" />
<a :href="myUrl">Move to url</a>
</div>
<script>
const { createApp, ref } = Vue;
const app = createApp({
setup() {
const imageSrc = ref("https://picsum.photos/200");
const myUrl = ref("https://www.google.co.kr/");
return {
imageSrc,
myUrl,
};
},
});
app.mount("#app");
</script>
</body>
- 대괄호로 감싸서 argument에 Javascript 표현식을 사용할 수 있다.
- 대괄호 안에 작성하는 이름은 반드시 소문자로만 구성 가능하다. (브라우저가 속성 이름을 소문자로 강제 변환)
- Javascript 표현식에 따라 동적으로 평가된 값이 최종 argument 값으로 사용된다.
<body>
<div id="app">
<p :[dynamicattr]="dynamicValue">Dynamic Attr</p>
</div>
<script>
const { createApp, ref } = Vue;
const app = createApp({
setup() {
const dynamicattr = ref("title");
const dynamicValue = ref("Hello Vue.js");
const size = ref(50);
return {
dynamicattr,
dynamicValue,
};
},
});
app.mount("#app");
</script>
</body>
2. Class and Style Bindings
- 클래스와 스타일 모두 속성이므로 v-bind를 사용하여 문자열 값을 할당할 수 있음
- 그러나 번거롭고 오류가 발생하기 쉬움
- 객체 또는 배열을 활용한 개선 사항 제공
Class 바인딩
1) 객체를 활용한 바인딩
- 객체를 :class에 전달하여 클래스를 동적으로 전환
- 예시 1) isActive의 T/F에 의해 active 클래스의 존재가 결정됨
<body>
<div id="app">
<div :class="{ active: isActive }">Text1</div>
</div>
<script>
const { createApp, ref } = Vue;
const app = createApp({
setup() {
const isActive = ref(false);
return {
isActive,
};
},
});
app.mount("#app");
</script>
</body>
- 객체에 더 많은 필드를 포함하여 여러 클래스를 전환할 수 있음
- 예시 2) :class directive를 일반 클래스 속성과 함께 사용 가능
<body>
<div id="app">
<div class="static" :class="{ active: isActive, 'text-primary': hasInfo }">Text2</div>
<div class="static" :class="classObj">Text3</div>
</div>
<script>
const { createApp, ref } = Vue;
const app = createApp({
setup() {
const isActive = ref(false);
const hasInfo = ref(true);
// ref는 반응 객체의 속성으로 액세스되거나 변경될 때 자동으로 래핑 해제
const classObj = ref({
active: isActive,
"text-primary": hasInfo,
});
return {
isActive,
hasInfo,
classObj,
};
},
});
app.mount("#app");
</script>
</body>
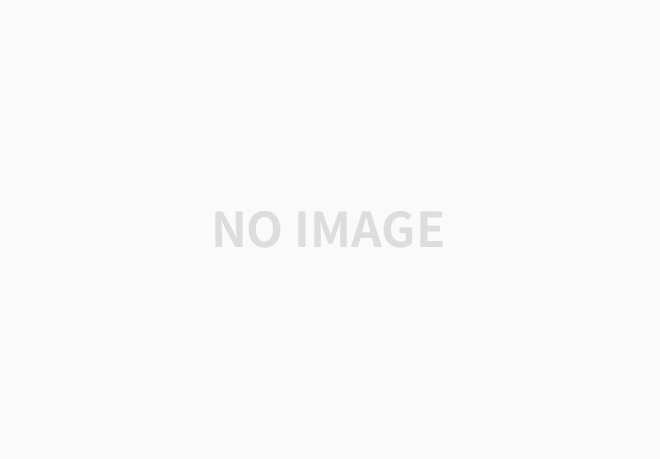
2) 배열을 활용한 바인딩
- :class를 배열에 바인딩하여 클래스 목록을 적용할 수 있음
- 배열 내에서 객체 구문 사용
<body>
<div id="app">
<div :class="[activeClass, infoClass]">Text4</div>
<div :class="[{ active: isActive }, infoClass]">Text5</div>
</div>
<script>
const { createApp, ref } = Vue;
const app = createApp({
setup() {
const isActive = ref(false);
const activeClass = ref("active");
const infoClass = ref("text-primary");
return {
activeClass,
infoClass,
};
},
});
app.mount("#app");
</script>
</body>
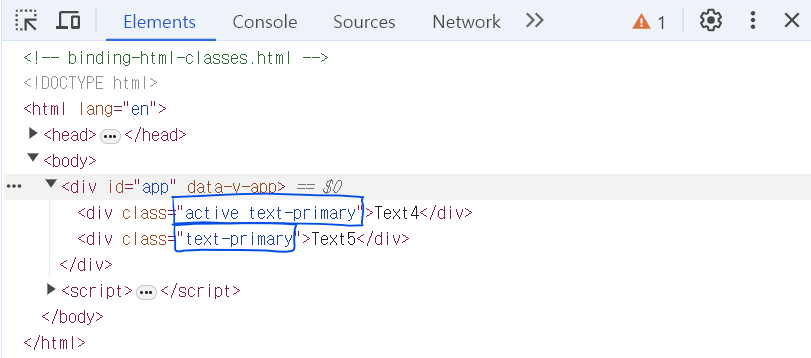
Style 바인딩
1) 객체를 활용한 바인딩
- :style은 Javascript 객체 값에 대한 바인딩을 지원한다.
<body>
<div id="app">
<div :style="{ color: activeColor, fontSize: fontSize + 'px' }">Text</div>
<div :style="{ 'font-size': fontSize + 'px' }">Text</div>
</div>
<script>
const { createApp, ref } = Vue
const app = createApp({
setup() {
const activeColor = ref("crimson")
const fontSize = ref(50)
return {
activeColor,
fontSize,
}
},
})
app.mount("#app")
</script>
</body>
- 스타일 객체에 직접 바인딩하여 템플릿을 더 깔끔하게 작성할 수 있다.
<body>
<div id="app">
<div :style="styleObj">Text</div>
</div>
<script>
const { createApp, ref } = Vue
const app = createApp({
setup() {
const activeColor = ref("crimson")
const fontSize = ref(50)
const styleObj = ref({
color: activeColor.value,
fontSize: fontSize.value + "px",
})
return {
styleObj,
}
},
})
app.mount("#app")
</script>
</body>
2) 배열을 활용한 바인딩
<body>
<div id="app">
<div :style="[styleObj, styleObj2]">Text</div>
</div>
<script>
const { createApp, ref } = Vue
const app = createApp({
setup() {
const activeColor = ref("crimson")
const fontSize = ref(50)
const styleObj = ref({
color: activeColor.value,
fontSize: fontSize.value + "px",
})
const styleObj2 = ref({
color: "blue",
border: "1px solid black",
})
return {
activeColor,
fontSize,
styleObj,
styleObj2,
}
},
})
app.mount("#app")
</script>
</body>
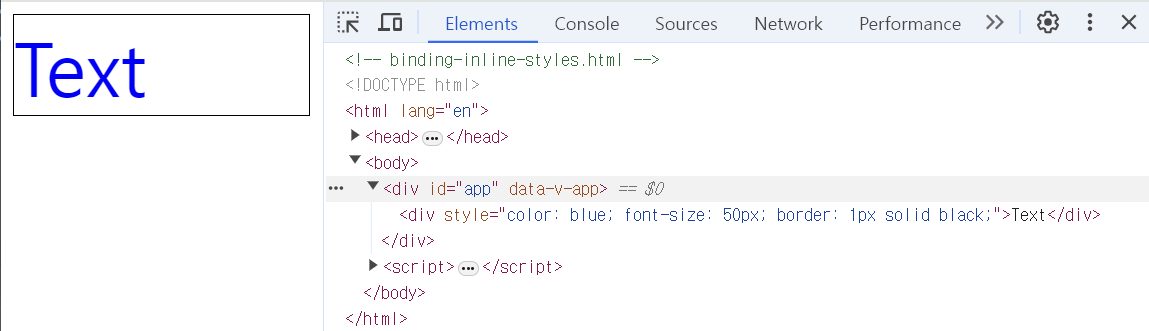
728x90
반응형
'Frontend > Vue3' 카테고리의 다른 글
[Vue3] Computed Property (0) | 2024.05.06 |
---|---|
[Vue3] Form 입력 바인딩 (v-model) (0) | 2024.05.05 |
[Vue3] 이벤트 핸들링 (v-on) (0) | 2024.05.05 |
[Vue3] 템플릿 문법 (0) | 2024.05.04 |
[Vue3] Vite를 이용하여 Vue 프로젝트 생성하기 (0) | 2024.05.04 |